What is Sitemap ?
A sitemap is a file that lists the pages of a website to inform search engines about the organization of its content. It typically includes information such as URLs, metadata about each URL (such as when it was last updated, how often it changes, and its priority relative to other URLs on the site), and other details that help search engines crawl and index the site more effectively.
Lets to create a create a virtual environment
python -m venv venv
.\venv\Scripts\activate
python -m pip install --upgrade pip
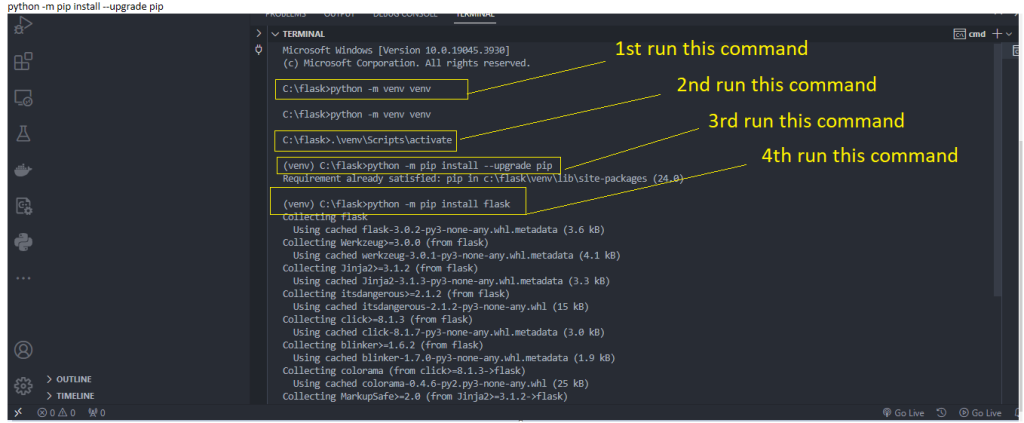
Next to install the flask app
pip install flask
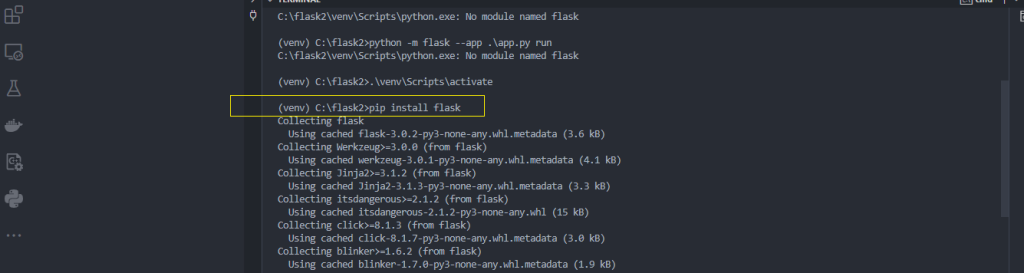
Next to below file and put the code
app.py
import sys
import logging
import asyncio
import os
from flask import Flask, render_template, request, send_file
from pysitemap import crawler
app = Flask(__name__)
def run_crawler(root_url):
try:
# Set up event loop
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
if "--iocp" in sys.argv:
logging.info('using iocp')
el = asyncio.ProactorEventLoop()
asyncio.set_event_loop(el)
# Run crawler and generate sitemap
crawler(root_url, out_file='sitemap.xml')
return True # Indicate successful crawling
except Exception as e:
app.logger.error(f'Error generating sitemap: {str(e)}')
return False # Indicate crawling failed
@app.route('/')
def index():
return render_template('index.html', show_download_button=False)
@app.route('/generate_sitemap', methods=['POST'])
def generate_sitemap():
root_url = request.form.get('url')
if root_url:
success = run_crawler(root_url)
if success:
return render_template('index.html', show_download_button=True)
else:
return render_template('index.html', show_download_button=False, error='Error generating sitemap')
else:
return render_template('index.html', show_download_button=False, error='Invalid URL')
@app.route('/download_sitemap')
def download_sitemap():
try:
sitemap_path = os.path.join(os.getcwd(), 'sitemap.xml')
return send_file(sitemap_path, as_attachment=True)
except Exception as e:
app.logger.error(f'Error downloading sitemap: {str(e)}')
return f'Error downloading sitemap: {str(e)}'
if __name__ == '__main__':
app.run(debug=True)
Next to create new folder name is templates/index.html and paste below code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sitemap Generator</title>
<style>
/* CSS for the processing message */
#processing-message {
display: none;
}
</style>
</head>
<body>
<h1>Sitemap Generator</h1>
<form id="generate-form" action="/generate_sitemap" method="post">
<input type="text" name="url" placeholder="Enter URL">
<button id="generate-button" type="submit">Generate Sitemap</button>
</form>
<!-- Processing message -->
<div id="processing-message">
<p>Generating sitemap. Please wait...</p>
</div>
<!-- Download button -->
<div id="download-button" style="display: none;">
<a href="/download_sitemap" download><button>Download Sitemap</button></a>
</div>
<!-- Error message -->
{% if error %}
<p>{{ error }}</p>
{% endif %}
<script>
// Function to show processing message and hide form
function showProcessing() {
document.getElementById('generate-form').style.display = 'none';
document.getElementById('processing-message').style.display = 'block';
}
// Function to hide processing message and show download button
function showDownloadButton() {
document.getElementById('processing-message').style.display = 'none';
document.getElementById('download-button').style.display = 'block';
}
// Event listener for form submission
document.getElementById('generate-form').addEventListener('submit', function() {
showProcessing(); // Show processing message when form is submitted
});
// Event listener for showing download button
{% if show_download_button %}
showDownloadButton(); // Show download button if available
{% endif %}
</script>
</body>
</html>
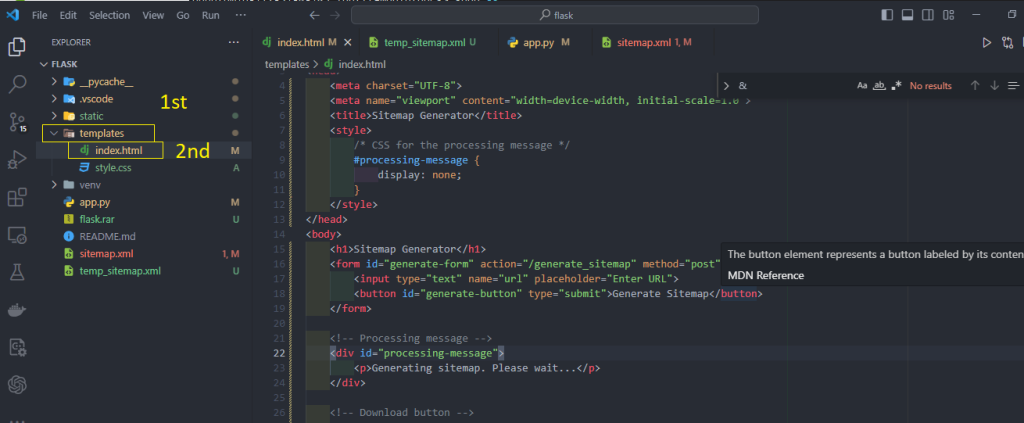
Now run the command
python -m flask --app .\app.py run
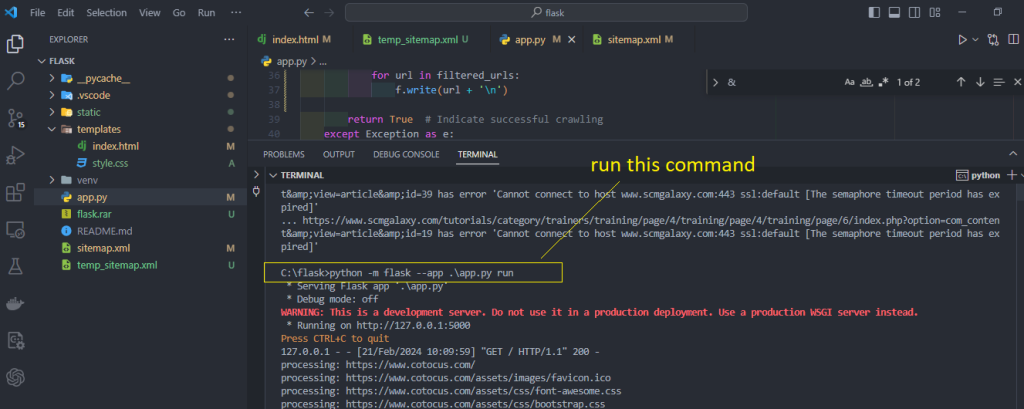
Next to open development server and check
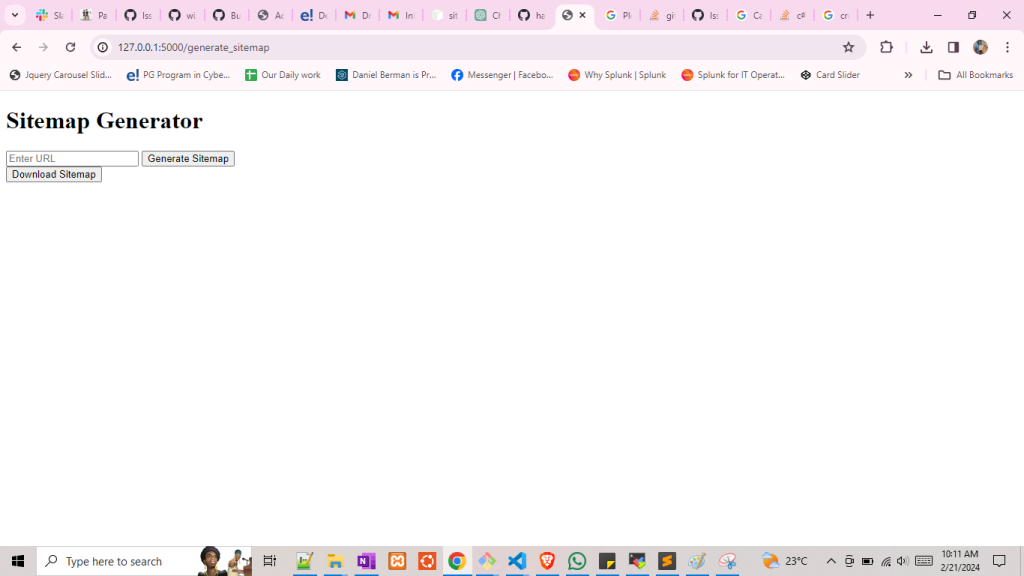