In this tutorial i going to share you top 10 Security Checklist for laravel for important for security purpose.
1. CSRF Protection
What is CSRF ?
A CSRF token in Laravel is a unique value that is generated for each user session and used to prevent cross-site request forgery (CSRF) attacks. CSRF attacks are a type of malicious exploit whereby unauthorized commands are performed on behalf of an authenticated user.
Why We need to do CSRF Protection ?
CSRF protection is necessary because it can prevent attackers from performing unauthorized actions on behalf of authenticated users. This can include actions such as transferring money, changing passwords, or sending emails.
- Unauthorized Actions: CSRF attacks can lead to users unknowingly performing actions on a website without their consent. This could include changing account settings, making financial transactions, or performing other sensitive operations.
- Data Integrity: CSRF attacks can compromise the integrity of user data. Attackers might manipulate data or settings, leading to undesirable consequences for both users and the website.
Security thread if i ignore CSRF Protection
If you ignore CSRF protection, your web application will be vulnerable to cross-site request forgery (CSRF) attacks. CSRF attacks are a type of malicious exploit whereby unauthorized commands are performed on behalf of an authenticated user.
CSRF attacks can be used to perform a variety of malicious actions, such as:
- Changing passwords
- Sending emails
- Deleting files
Example :-
<form method="POST" action="#">
@csrf
<label class="control-label">Name</label>
<input type="text" name="name" id="name" class="form-control" required
autocomplete="off" />
<label class="control-label">Email Address</label>
<input type="text" name="email_address" id="email_address" class="form-control" required
autocomplete="off" />
<button type="submit">Submit</button>
</form>
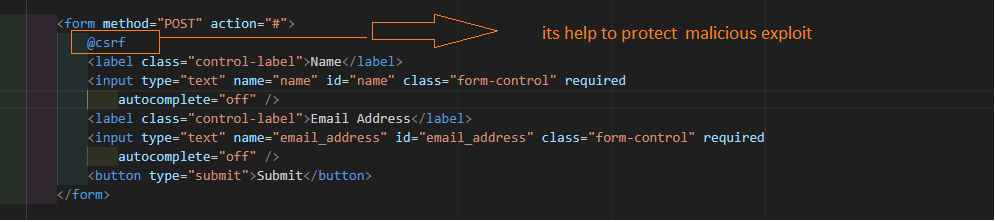
2. Cookies Protection
What is Cookies ?
Cookies in Laravel are small pieces of data that are stored in the user’s web browser and used to identify the user or track their activity on a website. Laravel provides a number of features to make it easy to manage cookies in your application.
Why We need to do Cookies ?
Cookies in Laravel serve various purposes and are essential for building dynamic and interactive web applications. Here are some reasons why cookies are commonly used in Laravel:
- Flash Messages:
- Flash messages, which are messages that persist only for the next request, are often stored in cookies. They allow developers to display feedback or notifications to users after a specific action.
- User Authentication:
- Cookies are often used to store authentication information. After a user logs in, a secure, encrypted cookie can be set to maintain the user’s authenticated state between requests.
Security thread if i ignore Cookies Protection
If you ignore cookies protection, your web application will be vulnerable to a number of security threats, including:
- Session hijacking: An attacker could steal a user’s session cookie and use it to impersonate the user on your website. This could allow the attacker to access the user’s account, make unauthorized purchases, or send malicious messages on the user’s behalf.
- Cross-site scripting (XSS) attacks: An XSS attack occurs when an attacker injects malicious code into a web page. If a user visits a web page that has been infected with XSS code, the code can be executed in the user’s browser and could allow the attacker to steal the user’s cookies.
- Cookie theft: An attacker could steal a user’s cookies using a variety of methods, such as phishing attacks or malware. Once an attacker has stolen a user’s cookies, they can use them to impersonate the user on your website or access other websites that the user has logged into.
Example :-
<?php
namespace App\Http\Controllers;
use Illuminate\Support\Facades\Cookie;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Log;
class LearningController extends Controller
{
public function index()
{
$value = cookie('example_cookie');
Log::info("message me ky aa arh ahi".$value);
return view('learning',compact('value'));
}
}
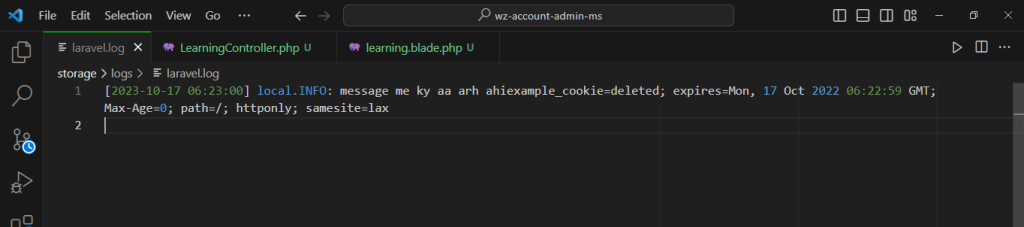
3. Disable debug messages in production
What is debug message ?
A debug message in Laravel is a message that is displayed to the developer to help them debug their code. Debug messages can be used to log information about the state of the application, such as the values of variables or the output of function calls. Debug messages can also be used to display error messages or warnings.
Why We need to do debug message ?
We need to do debug messages in Laravel to help us identify and fix bugs in our code. Debug messages can be used to log information about the state of the application, such as the values of variables or the output of function calls. They can also be used to display error messages or warnings.
Security thread if i ignore this debug message
If you ignore debug messages in Laravel, you may miss important information about the state of your application and potential security threats. This can make it more difficult to identify and fix bugs
Example :-
Go to .env file and do as below
APP_DEBUG=false
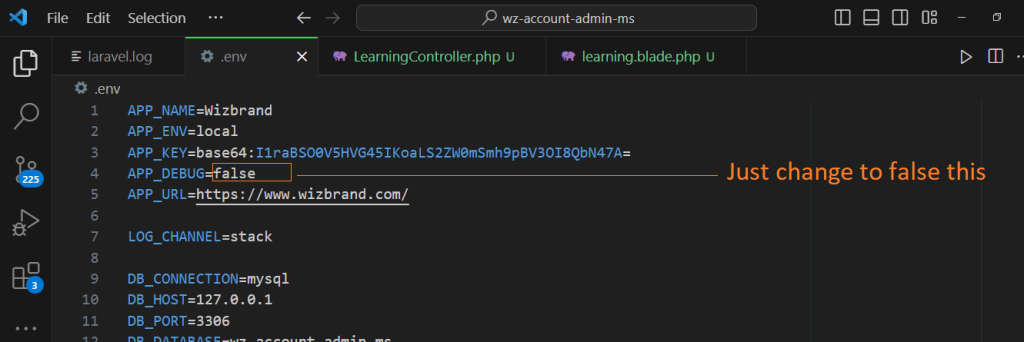
4. Input Validation
Never trust user input, and always validate data before using it in your application. Laravel’s validation features make this straightforward, allowing you to apply validation rules to your incoming data easily.
$request->validate([
'title' => 'required|unique:posts|max:255',
'body' => 'required',
]);
5. User Authentication
Use CAPTCHA for any endpoints that can be exploited using brute-force techniques. This includes login, registration, and forgot password forms. CAPTCHA will stop most automated attacks. Go with something like Google’s reCAPTCHA rather than developing your own implementation.
Build multi-factor authentication for your member and admin accounts. There are great packages available that you can use to generate QR codes and validate one-time password codes upon login. Avoid other means of delivering this code, such as email or SMS. It simply isn’t secure enough.
Security thread if i ignore Google Capthca
you ignore Google reCAPTCHA in your Laravel application, you may be exposing your application to a number of security threats, including:
- Account takeover (ATO): ATO is a type of attack where an attacker gains control of a user’s account. Attackers can use ATO to steal sensitive data, such as passwords, credit card numbers, and personal information.
- Spam: Attackers can use your application to send spam emails to your users. This can damage your reputation and make your users less likely to trust your application.
- Denial-of-service (DoS): DoS attacks are designed to overwhelm your application with traffic, making it unavailable to legitimate users.
- Fraud: Attackers can use your application to commit fraud, such as creating fake accounts or making unauthorized purchases.
6. Password hashing
What is password hashing ?
Password hashing in Laravel is the process of converting a user’s password into a secure, one-way hash. This hash is then stored in the database instead of the plain text password. This makes it much more difficult for attackers to steal user passwords, even if they are able to gain access to the database.
Why We need to do this password hashing ?
Password hashing is a crucial security measure, and implementing it in Laravel or any web application is essential for several reasons:
- Protection Against Data Breaches:
- In the unfortunate event of a database breach, hashed passwords significantly reduce the impact. Hashing ensures that even if the hashed passwords are exposed, attackers cannot easily reverse the process to obtain the original passwords.
- User Privacy:
- Hashing passwords protects user privacy by preventing the exposure of their actual passwords. Users often reuse passwords across multiple services, and protecting their passwords adds an extra layer of security.
- Compliance with Best Practices:
- It is considered a best practice and a standard in web security to hash passwords. Following established security practices helps ensure that your application meets industry standards and expectations.
- Mitigation of Credential Stuffing Attacks:
- Hashing passwords helps mitigate credential stuffing attacks where attackers use known username and password combinations obtained from other breaches to gain unauthorized access to user accounts.
- Authentication Security:
- Hashing ensures the security of the authentication process. When users log in, their entered passwords are hashed and compared to the stored hashed passwords. This allows for secure user authentication without exposing sensitive information.
- Protection Against Insider Threats:
- Even in scenarios where an insider with access to the database tries to misuse their privileges, hashed passwords make it more challenging for them to abuse user credentials.
Security thread if i ignore Password Hashing ?
- Account takeover (ATO): ATO is a type of attack where an attacker gains control of a user’s account. Attackers can use ATO to steal sensitive data, such as passwords, credit card numbers, and personal information.
- Password breaches: If an attacker gains access to your database, they can steal all of your users’ passwords in plain text. This can lead to a massive password breach that can affect millions of users.
- Rainbow tables: Rainbow tables are pre-computed tables that can be used to crack hashed passwords. If an attacker has access to a rainbow table, they may be able to crack your users’ passwords, even if you are using a strong hashing algorithm.
Example :-
$check_user_email->password = Hash::make($request['password']);
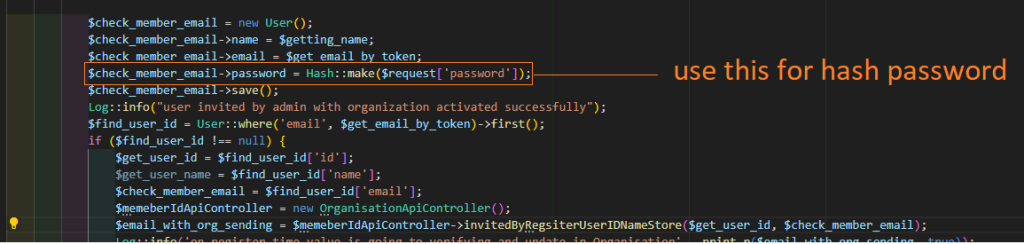
7. Use Encryption
In laravel use encryption and decryption for store the private information, leverages the OpenSSL library to provide AES-256 and AES-128 encryption. To ensure that no encrypted data can be modified by an unauthorized party, Laravel signs encrypted values using a Message Authentication Key.
Why We need to do Encryption in laravel ?
We need to do encryption in Laravel to protect sensitive data from unauthorized access. Encryption is the process of converting data into a format that is unreadable without the proper decryption key. This makes it much more difficult for attackers to steal sensitive data, even if they are able to gain access to the database or other storage location.
Security thread if i ignore Encryption in laravel ?
If you ignore encryption in Laravel, you will be exposing your application to a number of security threats, including:
- Data breaches: If an attacker gains access to your database, they will be able to steal all of your users’ sensitive data, such as passwords, credit card numbers, and personal information. This can lead to a massive data breach that can affect millions of users.
- Compliance violations: If you are required to comply with industry regulations, such as PCI DSS and HIPAA, failing to encrypt sensitive data can lead to compliance violations. This can result in fines, penalties, and other legal consequences.
- Fraud: Attackers can use stolen sensitive data to commit fraud, such as making unauthorized purchases or creating fake accounts.
- Identity theft: Attackers can use stolen sensitive data to commit identity theft, such as opening new accounts in the victim’s name or taking out loans in their name.
Example :-
$encryptedMessage = Crypt::encrypt('This is a secret message');
$decryptedMessage = Crypt::decrypt($encryptedMessage);
8. Expiring and destroying HTTP sessions
What is Http Session ?
An HTTP session in Laravel is a way to store data about a user’s activity on your website across multiple HTTP requests. This can be useful for storing information such as the user’s login status, shopping cart items, or recently viewed pages.
Why We need to do destroying HTTP sessions ?
We need to destroy HTTP sessions in Laravel to improve security and performance.
Security:
- Prevent session hijacking: Session hijacking is a type of attack where an attacker steals a user’s session cookie and uses it to impersonate the user on your application. By destroying sessions when they are no longer needed, you can make it more difficult for attackers to hijack sessions.
- Prevent unauthorized access to session data: Session data can contain sensitive information about the user, such as their login status, shopping cart items, or recently viewed pages. By destroying sessions when they are no longer needed, you can prevent unauthorized access to this data.
- Prevent session fixation: Session fixation is a type of attack where an attacker forces a user to use a specific session ID. This can be done by exploiting a vulnerability in your application or by tricking the user into clicking on a malicious link. By destroying sessions when they are no longer needed, you can prevent session fixation attacks.
Example:-
public function logout()
{
session()->expireNow();
session()->invalidate();
return redirect()->route('home');
}
This code will expire and destroy the current session and then redirect the user to the home page.
9. Using Laravel’s Authentication and Authorization
What is Authentication and Authorization in laravel ?
Authentication in Laravel is the process of verifying that a user is who they say they are. Authorization is the process of determining whether a user is allowed to perform a given action.
Laravel provides a number of features to make it easy to implement authentication and authorization in your applications.
Authentication
Laravel provides a number of authentication drivers, including database, Eloquent, and LDAP. By default, Laravel uses the database authentication driver.
Authorization
Laravel provides a number of ways to authorize users. One way is to use Laravel’s built-in gates. Gates are functions that can be used to determine whether a user is authorized to perform a given action.
Security thread if i ignore Authentication and Authorization ?
If you ignore authentication and authorization in Laravel, you will be exposing your application to a number of security threats, including:
- Unauthorized access to sensitive data: Sensitive data, such as user data, financial data, and trade secrets, can be accessed by unauthorized users if you ignore authentication and authorization.
- Data breaches: Data breaches can occur if unauthorized users are able to access sensitive data.
- Denial-of-service (DoS): DoS attacks can be used to overwhelm your application with traffic, making it unavailable to legitimate users.
- Fraud: Attackers can use your application to commit fraud, such as creating fake accounts or making unauthorized purchases.
Example :-
$user = Auth::user();
Route::get('/admin', function () {
// Check if the user is authenticated.
if (! Auth::check()) {
// The user is not authenticated. Redirect them to the login page.
return redirect('/login');
}
// The user is authenticated. Show them the admin page.
return view('admin');
})->middleware('auth');
10 . Validate User Input
User input validation in Laravel refers to the process of verifying and ensuring that the data submitted by users through forms or other input mechanisms adheres to predefined rules and criteria. Laravel provides a robust validation system that allows developers to define and enforce rules for validating user input, reducing the risk of security vulnerabilities and enhancing the overall reliability of the application.
Security thread if i ignore User Input in Laravel ?
If you ignore user input in Laravel, you will be exposing your application to a number of security threats, including:
- Cross-Site Scripting (XSS): XSS is a type of attack where an attacker injects malicious code into a web page. This code can then be executed by the victim’s browser, giving the attacker control of the victim’s account or session.
- SQL Injection: SQL injection is a type of attack where an attacker injects malicious SQL code into a database query. This code can then be executed by the database, allowing the attacker to steal data, modify data, or delete data.
- Remote Code Execution (RCE): RCE is a type of attack where an attacker executes arbitrary code on a victim’s machine. This can be done by exploiting a vulnerability in a web application or by tricking the victim into downloading and executing a malicious file.
Example :-
$validatedData = $request->validate([
'login' => 'required|email',
'password' => 'required|min:8',
]);
$validatedData = $request->validate([
'name' => 'required|string',
'email' => 'required|email',
]);
[…] Top 10 Security Checklist for laravel ? […]